使用 Object.create 建立多層繼承
這邊我們先看到基本的層級 ↓
1 2 3 4 5 6 7 8 9 10 11 12
| var a = []; // Object > Array > a(實體)
function Dog(name, color, size) { this['name'] = name; this['color'] = color; this['size'] = size; };
var Bibi = new Dog('比比', '棕色', '小'); console.log(Bibi); // Object > Dog > Bibi(實體)
|
如果我們想要在Dog
前面新增一個層級Object > Animal > Dog
要怎麼做呢?
Object.create() 如何運用?
1 2 3 4 5 6 7 8 9 10 11 12 13
| var Bibi = { name: '比比', color: '棕色', size: '小', bark: function(){ console.log(this.name + ' 吠叫'); } };
var pupu = Object.create(Bibi); pupu.name = '噗噗'; console.log(pupu); console.log(pupu.bark());
|
會出現 ↓
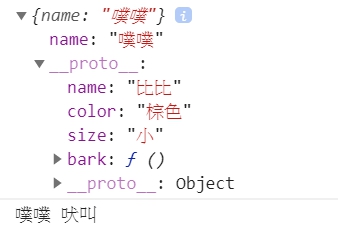
這邊可以發現 pupu
完全繼承了 Bibi
的所有屬性及方法。
我們在創造多層的原型鍊時,就會使用到 Object.create
的這個方法。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| function Animal(family) { this.kingdom = '動物界'; this.family = family || '人科'; };
Animal.prototype.move = function (e) { console.log(this.name + ' 移動'); };
function Dog(name, color, size) { this['name'] = name; this['color'] = color; this['size'] = size; };
// 使用 Object.create() 讓 Dog 繼承在 Animal 身上 Dog.prototype = Object.create(Animal.prototype); Dog.prototype.bark = function (e) { console.log(this.name + ' 吠叫'); }
var Bibi = new Dog('比比', '棕色', '小'); console.log(Bibi); Bibi.bark(); Bibi.move();
|
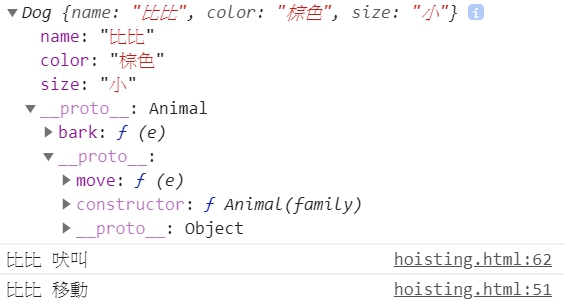
這邊還缺少了分類的部分,如果查詢 Bibi
的 family
會發現是 undefined
,所以現在我們來新增一下科別!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| function Animal(family) { this.kingdom = '動物界'; this.family = family || '人科'; };
Animal.prototype.move = function (e) { console.log(this.name + ' 移動'); };
function Dog(name, color, size) { // 利用 call 指定 this 能夠指向每一個實體 Animal.call(this, '犬科'); this['name'] = name; this['color'] = color; this['size'] = size; };
// 使用 Object.create() 讓 Dog 繼承在 Animal 身上 Dog.prototype = Object.create(Animal.prototype); Dog.prototype.bark = function (e) { console.log(this.name + ' 吠叫'); }
var Bibi = new Dog('比比', '棕色', '小'); console.log(Bibi); Bibi.bark(); Bibi.move();
|
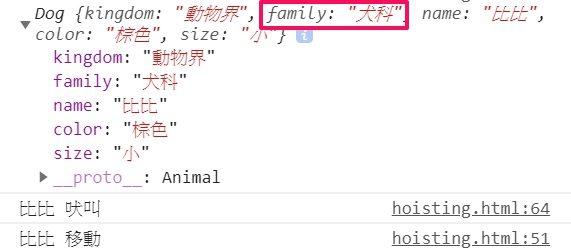
這樣的原型鍊繼承其實還是沒有很完整喔!我們會發現Dog
少了constructor
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| function Animal(family) { this.kingdom = '動物界'; this.family = family || '人科'; };
Animal.prototype.move = function (e) { console.log(this.name + ' 移動'); };
function Dog(name, color, size) { // 利用 call 指定 this 能夠指向每一個實體 Animal.call(this, '犬科'); this['name'] = name; this['color'] = color; this['size'] = size; };
// 使用 Object.create() 讓 Dog 繼承在 Animal 身上 Dog.prototype = Object.create(Animal.prototype); // 這裡使用 prototype 把 constructor 補上就沒問題囉 Dog.prototype.constructor = Dog; Dog.prototype.bark = function (e) { console.log(this.name + ' 吠叫'); }
var Bibi = new Dog('比比', '棕色', '小'); console.log(Bibi); Bibi.bark(); Bibi.move();
|
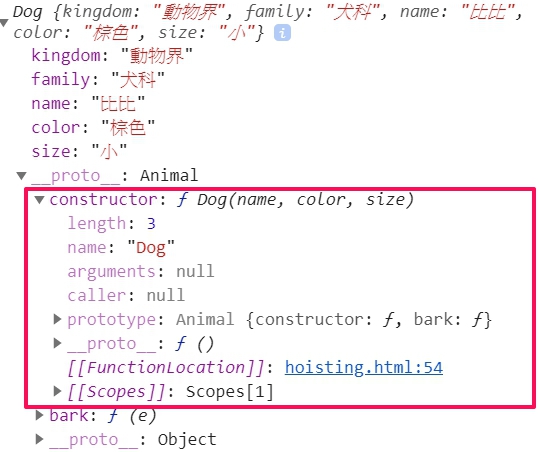