Getter 與 Setter,賦值運算不使用函式
setter - 存值的方法
1 2 3 4 5 6 7 8 9 10
| var wallet = { total: 100, set save (price){ this['total'] = this['total'] + price/2; } };
wallet['save'] = 300;
console.log(wallet['total']); // 250
|
wallet['save'] = 300;
這邊右邊的 300 會傳入 price
這個參數內。
這裡並不是用函式的方式來執行setter
,而是用賦值的方式。
getter - 取得特定值的方法
getter
因為是取值的概念,所以不會傳入參數
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| var wallet = { total: 100, set save(price) { this.total = this.total + price / 2; }, get save() { // 因為是取值所以要加入 return return this.total / 2; } };
console.log(wallet.save); wallet.save = 300; console.log(wallet);
|
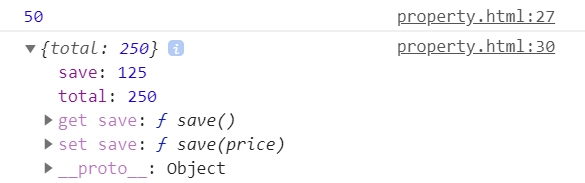
利用 Object.defineProperty()
來定義 Getter 與 Setter
除了上述的方法之外,利用Object.defineProperty()
也能夠設定 Getter 與 Setter。
1 2 3 4 5 6 7 8 9 10 11 12
| var wallet = { total: 100, };
Object.defineProperty(wallet, 'save', { set: function (price) { this.total = this.total + price / 2; }, get: function () { return this.total / 2; } })
|
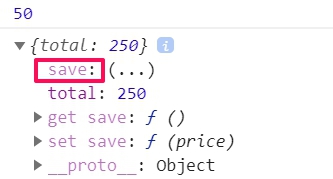
可以發現被紅色框框所框起來的地方顏色變了,這是因為屬性特徵上用defineProperty
定義出來它預設會是:不可刪除
、不可列舉
1 2
| // 後面加上這組程式碼來驗證看看 console.log(Object.getOwnPropertyDescriptor(wallet, 'save'));
|
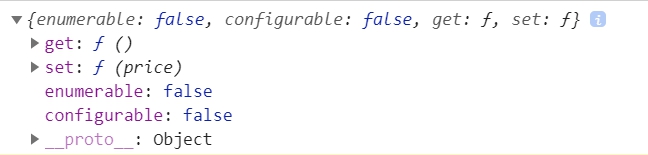
那這邊也有解決方法 ↓ ↓ ↓
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| var wallet = { total: 100, };
Object.defineProperty(wallet, 'save', { configurable: true, enumerable: true, set: function (price) { this.total = this.total + price / 2; }, get: function () { return this.total / 2; } })
|
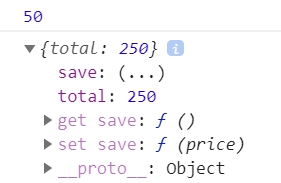
只要利用 defineProperty
把屬性特徵定義成「可以刪除」、「可以列舉」這樣就沒問題了,可以發現 save
的顏色也變回來了。